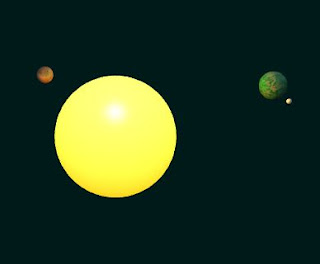
Recently I've started to experiment a bit with BabylonJS. Its nice WebGL framework for web. As an exercise I made this small example - moving and rotating solar system.
You can check the working example here - WebGL Solar System
Here is the full source code of the example: solar.js
var canvas; var engine; var scene; var sun; var planet1; var planet2; var planet2Moon; function start() { canvas = document.getElementById("renderCanvas"); engine = new BABYLON.Engine(canvas, true); scene = createScene(); engine.runRenderLoop(function () { scene.render(); }); var c = 0; var i = 0; scene.beforeRender = function () { planet1.position = new BABYLON.Vector3(20 * Math.sin(c), 0, 20 * Math.cos(c)); planet2.position = new BABYLON.Vector3(30 * Math.sin(i), 3 * Math.cos(i), 30 * Math.cos(i)); moon.position = new BABYLON.Vector3(5 * Math.sin(c), moon.parent.position.y, 5 * Math.cos(c)); c += 0.005; i += 0.008; }; window.addEventListener("resize", function () { engine.resize(); }); } var createScene = function () { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 0.1, 0.1); var camera = new BABYLON.ArcRotateCamera("ArcRotateCamera", 1, 0.8, 50, new BABYLON.Vector3(0, 0, 0), scene); camera.attachControl(canvas, false); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.5; light.diffuse = new BABYLON.Color3(1, 1, 1); var light0 = new BABYLON.PointLight("Omni0", new BABYLON.Vector3(1, 10, 1), scene); light0.diffuse = new BABYLON.Color3(1, 0.5, 0); light0.specular = new BABYLON.Color3(1, 1, 1); sun = BABYLON.Mesh.CreateSphere("sun", 20.0, 20.0, scene, false, BABYLON.Mesh.DEFAULTSIDE); var sunmaterial = new BABYLON.StandardMaterial('sunmaterial', scene); sunmaterial.diffuseColor = new BABYLON.Color3(1.00, 1.00, 1.00); sunmaterial.emissiveColor = new BABYLON.Color3(1.00, 0.72, 0.00); sunmaterial.ambientColor = new BABYLON.Color3(0.94, 0.85, 0.36); sunmaterial.specularColor = new BABYLON.Color3(1.00, 1.00, 1.00); sun.material = sunmaterial; planet1 = BABYLON.Mesh.CreateSphere("planet1", 10.0, 3.0, scene, false, BABYLON.Mesh.DEFAULTSIDE); var planet1mat = new BABYLON.StandardMaterial('planet1mat', scene); var planet1mat_diffuseTexture = new BABYLON.Texture('assets/planet1.png', scene); planet1mat.diffuseTexture = planet1mat_diffuseTexture; planet1mat.specularColor = new BABYLON.Color3(0, 0, 0); planet1.material = planet1mat; var frameRate = 10; var yRotate = new BABYLON.Animation("yRotate", "rotation.y", frameRate, BABYLON.Animation.ANIMATIONTYPE_FLOAT, BABYLON.Animation.ANIMATIONLOOPMODE_CYCLE); var keyFramesR = []; keyFramesR.push({ frame: 0, value: 0 }); keyFramesR.push({ frame: frameRate, value: Math.PI }); keyFramesR.push({ frame: 2 * frameRate, value: 2 * Math.PI }); yRotate.setKeys(keyFramesR); scene.beginDirectAnimation(planet1, [yRotate], 0, 2 * frameRate, true); planet2 = BABYLON.Mesh.CreateSphere("planet2", 10.0, 5.0, scene, false, BABYLON.Mesh.DEFAULTSIDE); moon = BABYLON.Mesh.CreateSphere("moon", 3.0, 1.0, scene, false, BABYLON.Mesh.DEFAULTSIDE); moon.translate(planet2.position, 5, BABYLON.Space.WORLD); moon.parent = planet2; var planet2mat = new BABYLON.StandardMaterial('planet2mat', scene); var planet2mat_diffuseTexture = new BABYLON.Texture('assets/planet2.jpg', scene); planet2mat.diffuseTexture = planet2mat_diffuseTexture; planet2mat.specularColor = new BABYLON.Color3(0, 0, 0); planet2.material = planet2mat; var yRotate = new BABYLON.Animation("yRotate", "rotation.y", frameRate/2, BABYLON.Animation.ANIMATIONTYPE_FLOAT, BABYLON.Animation.ANIMATIONLOOPMODE_CYCLE); yRotate.setKeys(keyFramesR); scene.beginDirectAnimation(planet2, [yRotate], 0, 2 * frameRate, true); return scene; };
No comments :
Post a Comment