Now lets continue with our game. In the init function remove the hello world code and add this row:
startGame();
and right afrer the init function we will add startGame:
private function startGame():void { this.addEventListener(Event.ENTER_FRAME, gameLoop); }And now the game loop:
private function gameLoop(event:Event = null):void { }
It is time to add the player. Lets make new folder called assets and add some images to it. For player spaceship I’ll use this cool image:
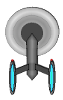
and for enemies this one:

You can use my images but please credit me.
Lets start with adding the player. More about embedding images you can learn from this post - FlashDevelop, ActionScript3 and images.
Add this in the class body:
[Embed(source="../assets/s1.png")] private var playerShipImage:Class; private var player:MovieClip;
And now alter the startGame function this way:
private function startGame():void { player = new MovieClip(); var bitmap:Bitmap = new playerShipImage(); bitmap.x = -bitmap.width / 2; bitmap.y = -bitmap.height / 2; player.addChild(bitmap); player.x = 250; player.y = 430; addChild(player); this.addEventListener(Event.ENTER_FRAME, gameLoop); }Compile and run! Here is the result:
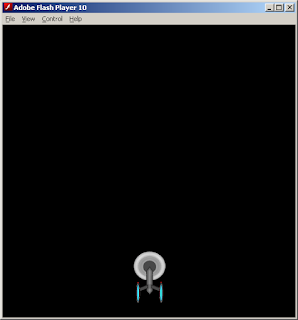
And here is the full source code till now:
Main.as
package { import flash.display.*; import flash.events.*; public class Main extends Sprite { [Embed(source="../assets/s1.png")] private var playerShipImage:Class; private var player:MovieClip; public function Main():void { if (stage) init(); else addEventListener(Event.ADDED_TO_STAGE, init); } private function init(e:Event = null):void { removeEventListener(Event.ADDED_TO_STAGE, init); // entry point startGame(); } private function startGame():void { player = new MovieClip(); var bitmap:Bitmap = new playerShipImage(); bitmap.x = -bitmap.width / 2; bitmap.y = -bitmap.height / 2; player.addChild(bitmap); player.x = 250; player.y = 430; addChild(player); this.addEventListener(Event.ENTER_FRAME, gameLoop); } private function gameLoop(event:Event = null):void { } } }Part 1