Just Another Bubble Shooter is a little bit different bubble shooter game with very fast gameplay. You can try the game here.
Here is the embed code:
Copy and paste this code to your site.
You can download the game from here SWF.
Friday, November 9, 2012
Monday, November 5, 2012
How to make simple shooting game like Balloon Hunt
For this tutorial I'll use only FlashDevelop as its easier this way. You can easily adapt it later to build with the Flash Ide.
So lets describe our game first. We will have flying targets all over the screen and we will click on them with the mouse in order to shoot them. You can play the original game here Balloon Hunt 2. First we will add the targets.
Now lets start with new AS3 project with size 640x480 and 50fps. Here is the stub code:
Now its time to add the action. We will add the function mouseDown:
Game.as
So lets describe our game first. We will have flying targets all over the screen and we will click on them with the mouse in order to shoot them. You can play the original game here Balloon Hunt 2. First we will add the targets.
Now lets start with new AS3 project with size 640x480 and 50fps. Here is the stub code:
package { import flash.display.*; import flash.utils.*; import flash.events.*; public class Game extends MovieClip { public function Game() { } } }Now I'll add an array for our targets:
private var targets:Array = new Array();And a separate class for our targets. Its a very simple class that extends MovieClip and we will use its graphics object to draw a circle in it.
import flash.display.*; public class Target extends MovieClip { public var hit:Boolean = false; public function Target() { graphics.beginFill(0xff0000); graphics.drawCircle(0, 0, 30); } }And a function to our game class to start the game:
import flash.display.*; import flash.utils.*; import flash.events.*; public class Game extends MovieClip { private var targets:Array = new Array(); public function Game() { startGame(); } private function startGame() : void { var target:Target = new Target(); target.x = 320; target.y = 240; this.addChild(target); this.addEventListener(Event.ENTER_FRAME, gameLoop); } private function gameLoop(e:Event) :void { } }Here you will see several things. First we add a target to our game just to see something on the screen. Next or actually first we call our function startGame from the constructor. And last we add a function that will be called every frame:
this.addEventListener(Event.ENTER_FRAME, gameLoop);This will be our game loop. Now you can run the project and see what we get - a lonely target stuck at the center of our game. Lets change this - we will add target on regular basis. We need a counter for this - targetCounter.
private var targetCounter:int; private const TARGETCOUNTER:int = 1000;And we will add the variable lastTime:
private var lastTime:int;And a code to the gameLoop function to track how much time have passed.
if(lastTime == 0) lastTime = getTimer(); var timeDiff:int = getTimer() - lastTime; lastTime += timeDiff;Here is the full code:
import flash.display.*; import flash.utils.*; import flash.events.*; public class Game extends MovieClip { private var targets:Array = new Array(); private var lastTime:int; private var targetCounter:int; private const TARGETCOUNTER:int = 500; public function Game() { startGame(); } private function startGame() : void { lastTime = 0; targetCounter = 0; this.addEventListener(Event.ENTER_FRAME, gameLoop); } private function gameLoop(e:Event) :void { if(lastTime == 0) lastTime = getTimer(); var timeDiff:int = getTimer() - lastTime; lastTime += timeDiff; } }Now we will start with adding targets and moving them around. I want to mention that the code is not optimized but I think it will be easier for beginners to understand it this way. Here is how we change the game loop now:
private function gameLoop(e:Event) :void { if(lastTime == 0) lastTime = getTimer(); var timeDiff:int = getTimer() - lastTime; lastTime += timeDiff; // next target counter targetCounter -= timeDiff; if (targetCounter <= 0) { // add new target var target:Target = new Target(); target.x = -30; target.y = Math.random() * 480; // random this.addChild(target); targets.push(target); targetCounter = TARGETCOUNTER; } // move all targets for (var i:int = targets.length - 1; i >= 0; i--) { var tg:Target = targets[i]; tg.x += timeDiff * 0.1; // check if the target is outside the screen if (tg.x > 670) { // 640 + 30 this.removeChild(tg); targets.splice(i, 1); } } }And here is the result:
Now its time to add the action. We will add the function mouseDown:
private function mouseDown(e:MouseEvent) { }And at startGame we will add this row:
this.addEventListener(MouseEvent.MOUSE_DOWN, mouseDown);And here is the full code of mouseDown:
private function mouseDown(e:MouseEvent):void { if (e.target is Target) { var target:Target = e.target as Target; target.hit = true; } }And now we must change the part where we check if we must remove a target:
if (tg.x > 670 || tg.hit) { // 640 + 30 this.removeChild(tg); targets.splice(i, 1); }And that's it all. Here is the full code if you've missed something:
Game.as
package { import flash.display.*; import flash.utils.*; import flash.events.*; public class Game extends MovieClip { private var targets:Array = new Array(); private var lastTime:int; private var targetCounter:int; private const TARGETCOUNTER:int = 1000; public function Game() { startGame(); } private function startGame() : void { lastTime = 0; targetCounter = 0; this.addEventListener(Event.ENTER_FRAME, gameLoop); this.addEventListener(MouseEvent.MOUSE_DOWN, mouseDown); } private function gameLoop(e:Event) :void { if(lastTime == 0) lastTime = getTimer(); var timeDiff:int = getTimer() - lastTime; lastTime += timeDiff; // next target counter targetCounter -= timeDiff; if (targetCounter <= 0) { // add new target var target:Target = new Target(); target.x = -30; target.y = Math.random() * 480; // random this.addChild(target); targets.push(target); targetCounter = TARGETCOUNTER; } // move all targets for (var i:int = targets.length - 1; i >= 0; i--) { var tg:Target = targets[i]; tg.x += timeDiff * 0.1; // check if the target is outside the screen if (tg.x > 670 || tg.hit) { // 640 + 30 this.removeChild(tg); targets.splice(i, 1); } } } private function mouseDown(e:MouseEvent):void { if (e.target is Target) { var target:Target = e.target as Target; target.hit = true; } } } }Target.as
package { import flash.display.*; public class Target extends MovieClip { public var hit:Boolean = false; public function Target() { graphics.beginFill(0x00ff00); graphics.drawCircle(0, 0, 30); graphics.endFill(); } } }And the final result:
Sunday, November 4, 2012
Balloon Hunt 2 - free shooting game for your website
Balloon Hunt 2 is fun family and kids friendly shooter game for your website or blog. Your goal is to hunt as many balloons as you can for 50 seconds and get the best possible highscore. You can try and play the game here - Balloon Hunt 2
Feel free to add it anywhere you want. Here is the embed code:
Copy this code and add it anywhere on your site. Or you can download the game from here - SWF Here are the thumbnails:
100x100 version
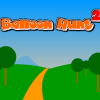
200x200 version
Feel free to add it anywhere you want. Here is the embed code:
Copy this code and add it anywhere on your site. Or you can download the game from here - SWF Here are the thumbnails:
100x100 version
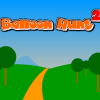
200x200 version
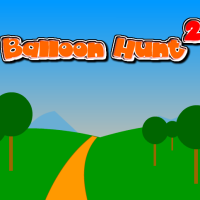
Saturday, June 23, 2012
Saturday, February 11, 2012
How to make space shooter game with ActionScript 3 and FlashDevelop - part 2
Part 1
Now lets continue with our game. In the init function remove the hello world code and add this row:
startGame();
and right afrer the init function we will add startGame:
It is time to add the player. Lets make new folder called assets and add some images to it. For player spaceship I’ll use this cool image:
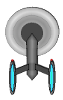
and for enemies this one:

You can use my images but please credit me.
Lets start with adding the player. More about embedding images you can learn from this post - FlashDevelop, ActionScript3 and images.
Add this in the class body:
And now alter the startGame function this way:
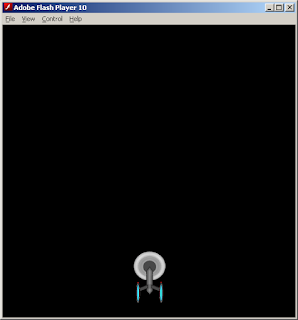
And here is the full source code till now:
Main.as
Now lets continue with our game. In the init function remove the hello world code and add this row:
startGame();
and right afrer the init function we will add startGame:
private function startGame():void { this.addEventListener(Event.ENTER_FRAME, gameLoop); }And now the game loop:
private function gameLoop(event:Event = null):void { }
It is time to add the player. Lets make new folder called assets and add some images to it. For player spaceship I’ll use this cool image:
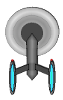
and for enemies this one:

You can use my images but please credit me.
Lets start with adding the player. More about embedding images you can learn from this post - FlashDevelop, ActionScript3 and images.
Add this in the class body:
[Embed(source="../assets/s1.png")] private var playerShipImage:Class; private var player:MovieClip;
And now alter the startGame function this way:
private function startGame():void { player = new MovieClip(); var bitmap:Bitmap = new playerShipImage(); bitmap.x = -bitmap.width / 2; bitmap.y = -bitmap.height / 2; player.addChild(bitmap); player.x = 250; player.y = 430; addChild(player); this.addEventListener(Event.ENTER_FRAME, gameLoop); }Compile and run! Here is the result:
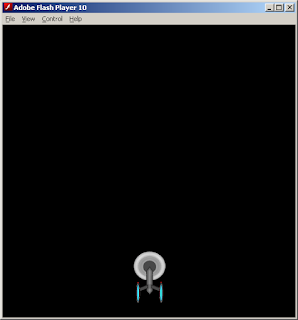
And here is the full source code till now:
Main.as
package { import flash.display.*; import flash.events.*; public class Main extends Sprite { [Embed(source="../assets/s1.png")] private var playerShipImage:Class; private var player:MovieClip; public function Main():void { if (stage) init(); else addEventListener(Event.ADDED_TO_STAGE, init); } private function init(e:Event = null):void { removeEventListener(Event.ADDED_TO_STAGE, init); // entry point startGame(); } private function startGame():void { player = new MovieClip(); var bitmap:Bitmap = new playerShipImage(); bitmap.x = -bitmap.width / 2; bitmap.y = -bitmap.height / 2; player.addChild(bitmap); player.x = 250; player.y = 430; addChild(player); this.addEventListener(Event.ENTER_FRAME, gameLoop); } private function gameLoop(event:Event = null):void { } } }Part 1
FlashDevelop and ActionScript 3 - how to embed image - fast tip
When you use the official flash ide, using images is as easy as clicking several buttons. Actually using images with FlashDevelop is even easier but if you haven't done it you will need this info.
First copy the desired image into folder of the project, for example I'll name my folder assets.
Add a variable to your class:
private var myImg:Class;
And place your cursor on the row above it. Right mouse button on the image and select Generate Embed Code:
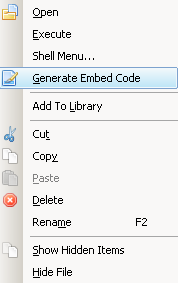
You will get something like this:
[Embed(source="../assets/s1.png")]
private var myImg:Class;
Add this where you want to use the image:
var bitmap:Bitmap = new myImg();
addChild(bitmap);
Easy, isn't it?
First copy the desired image into folder of the project, for example I'll name my folder assets.
Add a variable to your class:
private var myImg:Class;
And place your cursor on the row above it. Right mouse button on the image and select Generate Embed Code:
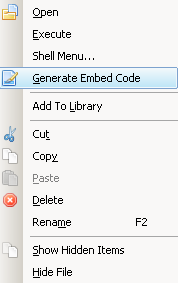
You will get something like this:
[Embed(source="../assets/s1.png")]
private var myImg:Class;
Add this where you want to use the image:
var bitmap:Bitmap = new myImg();
addChild(bitmap);
Easy, isn't it?
Wednesday, February 8, 2012
Typing Defense - free game for your website
Typing defense is fast paced typing game where you must defend your base from attacking enemy tanks.
You can play Typing Defense here.
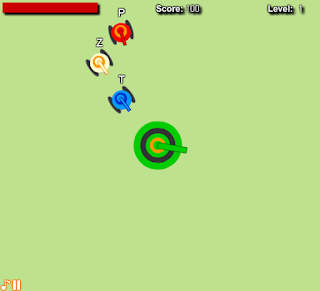
Embed Code:
You can download the game swf - Typing Defense SWF. Right button and choose save as.
Thumb:
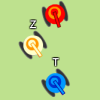
You can play Typing Defense here.
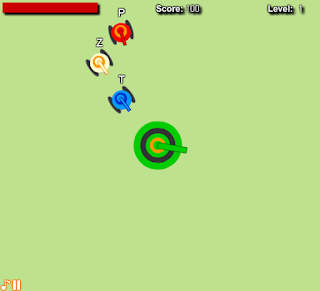
Embed Code:
You can download the game swf - Typing Defense SWF. Right button and choose save as.
Thumb:
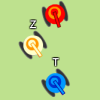
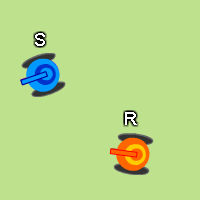
Tuesday, January 10, 2012
Get Cosmos Puzzle flash game free for your website
Cosmos Puzzle is relaxing match two game with space theme.
Get this game free for your website:
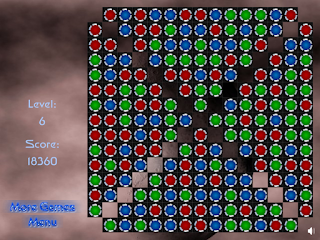
Embed Code:
Download SWF: Cosmos Puzzle
Thumb:
Get this game free for your website:
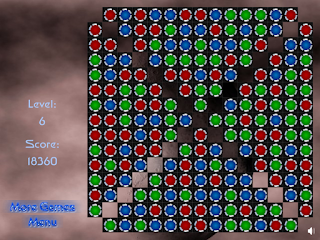
Embed Code:
Download SWF: Cosmos Puzzle
Thumb:
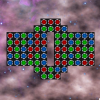
Saturday, January 7, 2012
Cute Cubes Collection - free game for your website
Get Cute Cubes Collection game free for your website:
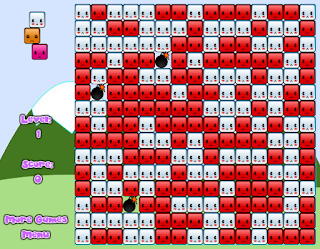
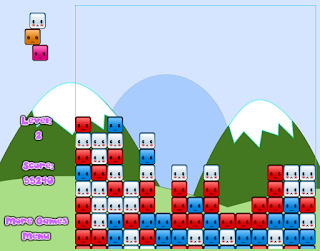
Embed Code:
Download game swf: Cute Cubes Collection
Thumb:
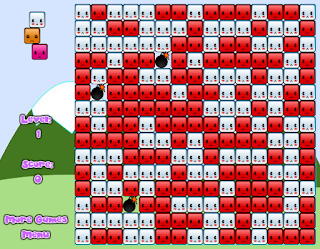
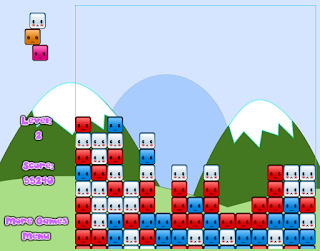
Embed Code:
Download game swf: Cute Cubes Collection
Thumb:
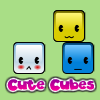
Subscribe to:
Posts
(
Atom
)